728x90
C++에서 두 수 a, b 중에 어떤 수가 더 큰지, 작은지 알고 싶다면
#include <algorithm>으로 <algorithm> 헤더를 추가하고
min(a, b);
또는
max(a, b);
이렇게 하면 된다.
하지만 배열이나 벡터의 원소들 중에서 최댓값을 찾고 싶다면 min_element, max_element 함수를 사용하면 된다. 이 함수들도 <algorithm> 라이브러리에 있기 때문에 <algorithm> 헤더를 포함하면 된다.
크기가 10인 배열 arr에서 최댓값을 찾아서 maxNum이라는 변수에 저장하고 싶다면
int maxNum = *max_element(arr, arr + 10);
이렇게 하면 된다. 앞에 역참조 연산자 *를 붙이는 이유는 max_element, min_element 함수의 반환값은 최댓값이 저장된 곳의 메모리 "주소"이기 때문이다. 따라서 값을 알고 싶다면 역참조연산자를 이용하면 된다.
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[10] = { 10, 200, 340, 28, 50, 189, 84, 39, 1, 248 };
cout << "최댓값: " << *max_element(arr, arr + 10) << endl;
cout << "최솟값: " << *min_element(arr, arr + 10) << endl;
return 0;
}
이 코드를 실행하면
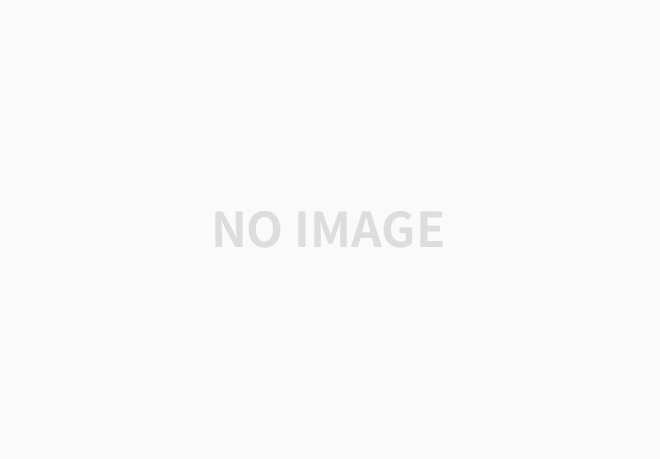
이렇게 배열 arr의 원소 중 최댓값과 최솟값을 알아낼 수 있다.
벡터도 마찬가지이다.
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> v = { 10, 200, 340, 28, 50, 189, 84, 39, 1, 248 };
cout << "최댓값: " << *max_element(v.begin(), v.end()) << endl;
cout << "최솟값: " << *min_element(v.begin(), v.end()) << endl;
return 0;
}
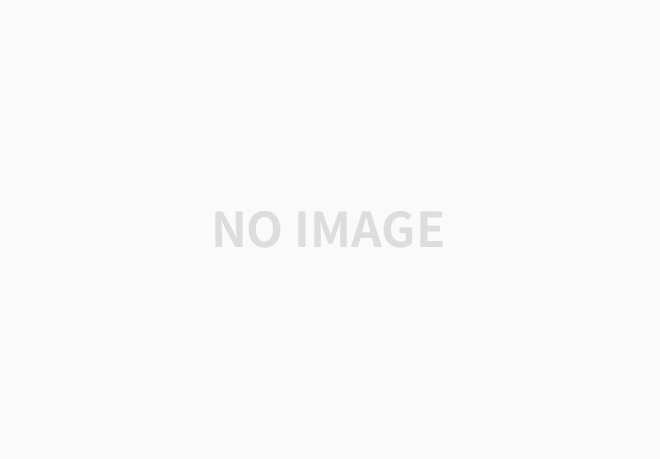
728x90
'C++' 카테고리의 다른 글
[C++] 벡터(vector)에서 특정 원소 삭제하는 법 (0) | 2020.04.11 |
---|---|
[C++] 포인터와 동적 메모리 할당 (포인터 심화2) (2) | 2020.03.31 |
[C++] 포인터와 배열 (포인터 심화) (0) | 2020.03.19 |
[C++] 배열 초기화, 벡터 초기화, fill 함수 (0) | 2020.03.14 |
[C++] next_permutation 이용해서 순열 구하기 (0) | 2020.02.19 |